Python3 面向对象
Python从设计之初就已经是一门面向对象的语言,正因为如此,在Python中创建一个类和对象是很容易的。本章节我们将详细介绍Python的面向对象编程。
如果你以前没有接触过面向对象的编程语言,那你可能需要先了解一些面向对象语言的一些基本特征,在头脑里头形成一个基本的面向对象的概念,这样有助于你更容易的学习Python的面向对象编程。
接下来我们先来简单的了解下面向对象的一些基本特征。
面向对象技术简介
- 类(Class): 用来描述具有相同的属性和方法的对象的集合。它定义了该集合中每个对象所共有的属性和方法。对象是类的实例。
- 类变量:类变量在整个实例化的对象中是公用的。类变量定义在类中且在函数体之外。类变量通常不作为实例变量使用。
- 数据成员:类变量或者实例变量用于处理类及其实例对象的相关的数据。
- 方法重写:如果从父类继承的方法不能满足子类的需求,可以对其进行改写,这个过程叫方法的覆盖(override),也称为方法的重写。
- 实例变量:定义在方法中的变量,只作用于当前实例的类。
- 继承:即一个派生类(derived class)继承基类(base class)的字段和方法。继承也允许把一个派生类的对象作为一个基类对象对待。例如,有这样一个设计:一个Dog类型的对象派生自Animal类,素以Dog也是一个Animal。
- 实例化:创建一个类的实例,类的具体对象。
- 方法:类中定义的函数。
- 对象:通过类定义的数据结构实例。对象包括两个数据成员(类变量和实例变量)和方法。
和其它编程语言相比,Python 在尽可能不增加新的语法和语义的情况下加入了类机制。
python3 类创建
面向对象编程是一种编程方式,此编程方式的落地需要使用 “类” 和 “对象” 来实现,所以,面向对象编程其实就是对 “类” 和 “对象” 的使用。
类就是一个模板,模板里可以包含多个函数,函数里实现一些功能
对象则是根据模板创建的实例,通过实例对象可以执行类中的函数
诶,你在这里是不是有疑问了?使用函数式编程和面向对象编程方式来执行一个“方法”时函数要比面向对象简便
- 面向对象:【创建对象】【通过对象执行方法】
- 函数编程:【执行函数】
观察上述对比答案则是肯定的,然后并非绝对,场景的不同适合其的编程方式也不同。
总结:函数式的应用场景 --> 各个函数之间是独立且无共用的数据
面向对象三大特性
面向对象的三大特性是指:封装、继承和多态。
一、封装
封装,顾名思义就是将内容封装到某个地方,以后再去调用被封装在某处的内容。
所以,在使用面向对象的封装特性时,需要:
- 将内容封装到某处
- 从某处调用被封装的内容
self 是一个形式参数,当执行 obj1 = Foo('wupeiqi', 18 ) 时,self 等于 obj1
当执行 obj2 = Foo('alex', 78 ) 时,self 等于 obj2
所以,内容其实被封装到了对象 obj1 和 obj2 中,每个对象中都有 name 和 age 属性,在内存里类似于下图来保存。
第二步:从某处调用被封装的内容
第二步:从某处调用被封装的内容
调用被封装的内容时,有两种情况:
- 通过对象直接调用
- 通过self间接调用
1、通过对象直接调用被封装的内容
上图展示了对象 obj1 和 obj2 在内存中保存的方式,根据保存格式可以如此调用被封装的内容:对象.属性名
2、通过self间接调用被封装的内容
执行类中的方法时,需要通过self间接调用被封装的内容
class Foo: def \_\_init\_\_(self, name, age):
self.name \= name
self.age \= age def detail(self): print(self.name) print(self.age)
obj1 \= Foo('chengd', 18)
obj1.detail() # Python默认会将obj1传给self参数,即:obj1.detail(obj1),所以,此时方法内部的 self = obj1,即:self.name 是 chengd ;self.age 是 18
obj2 \= Foo('python', 99)
obj2.detail() # Python默认会将obj2传给self参数,即:obj1.detail(obj2),所以,此时方法内部的 self = obj2,即:self.name 是 python ; self.age 是 99x
执行结果:
```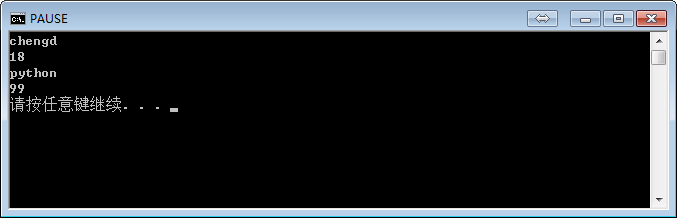
**综上所述,对于面向对象的封装来说,其实就是使用构造方法将内容封装到 对象 中,然后通过对象直接或者self间接获取被封装的内容。**
<table style="height: 225px; width: 1285px" dir="ltr" frame="void" rules="none" border="0" align="left"><tbody><tr><td><p><strong>练习二</strong>:游戏人生程序</p><p>1、创建三个游戏人物,分别是:</p><ul><li>苍井井,女,18,初始战斗力1000</li><li>东尼木木,男,20,初始战斗力1800</li><li>波多多,女,19,初始战斗力2500</li></ul><p>2、游戏场景,分别:</p><ul><li>草丛战斗,消耗200战斗力</li><li>自我修炼,增长100战斗力</li><li>多人游戏,消耗500战斗力</li></ul><div class="cnblogs_code"><img id="code_img_closed_c2a8d800-eb0b-4399-b0ae-1bc33b61f472" class="code_img_closed" src="https://img-hello-world.oss-cn-beijing.aliyuncs.com/add7401aa8cff5180d0b135db5f1dfef.gif" alt=""><img id="code_img_opened_c2a8d800-eb0b-4399-b0ae-1bc33b61f472" class="code_img_opened" style="display: none" src="https://img-hello-world.oss-cn-beijing.aliyuncs.com/0b676e2c5c2bc3f45ca0917cd49b2afe.gif" alt=""><div id="cnblogs_code_open_c2a8d800-eb0b-4399-b0ae-1bc33b61f472" class="cnblogs_code_hide"><pre><span style="color: rgba(0, 128, 0, 1)">#</span><span style="color: rgba(0, 128, 0, 1)"> -*- coding:utf-8 -*-</span>
<span style="color: rgba(0, 128, 0, 1)">#</span><span style="color: rgba(0, 128, 0, 1)"> ##################### 定义实现功能的类 #####################</span>
<span style="color: rgba(0, 0, 255, 1)">class</span><span style="color: rgba(0, 0, 0, 1)"> Person:
</span><span style="color: rgba(0, 0, 255, 1)">def</span> <span style="color: rgba(128, 0, 128, 1)">__init__</span><span style="color: rgba(0, 0, 0, 1)">(self, na, gen, age, fig):
self.name </span>=<span style="color: rgba(0, 0, 0, 1)"> na
self.gender </span>=<span style="color: rgba(0, 0, 0, 1)"> gen
self.age </span>=<span style="color: rgba(0, 0, 0, 1)"> age
self.fight </span>=<span style="color: rgba(0, 0, 0, 1)">fig
</span><span style="color: rgba(0, 0, 255, 1)">def</span><span style="color: rgba(0, 0, 0, 1)"> grassland(self):
</span><span style="color: rgba(128, 0, 0, 1)">"""</span><span style="color: rgba(128, 0, 0, 1)">注释:草丛战斗,消耗200战斗力</span><span style="color: rgba(128, 0, 0, 1)">"""</span><span style="color: rgba(0, 0, 0, 1)">
self.fight </span>= self.fight - 200
<span style="color: rgba(0, 0, 255, 1)">def</span><span style="color: rgba(0, 0, 0, 1)"> practice(self):
</span><span style="color: rgba(128, 0, 0, 1)">"""</span><span style="color: rgba(128, 0, 0, 1)">注释:自我修炼,增长100战斗力</span><span style="color: rgba(128, 0, 0, 1)">"""</span><span style="color: rgba(0, 0, 0, 1)">
self.fight </span>= self.fight + 200
<span style="color: rgba(0, 0, 255, 1)">def</span><span style="color: rgba(0, 0, 0, 1)"> incest(self):
</span><span style="color: rgba(128, 0, 0, 1)">"""</span><span style="color: rgba(128, 0, 0, 1)">注释:多人游戏,消耗500战斗力</span><span style="color: rgba(128, 0, 0, 1)">"""</span><span style="color: rgba(0, 0, 0, 1)">
self.fight </span>= self.fight - 500
<span style="color: rgba(0, 0, 255, 1)">def</span><span style="color: rgba(0, 0, 0, 1)"> detail(self):
</span><span style="color: rgba(128, 0, 0, 1)">"""</span><span style="color: rgba(128, 0, 0, 1)">注释:当前对象的详细情况</span><span style="color: rgba(128, 0, 0, 1)">"""</span><span style="color: rgba(0, 0, 0, 1)">
temp </span>= <span style="color: rgba(128, 0, 0, 1)">"</span><span style="color: rgba(128, 0, 0, 1)">姓名:%s ; 性别:%s ; 年龄:%s ; 战斗力:%s</span><span style="color: rgba(128, 0, 0, 1)">"</span> %<span style="color: rgba(0, 0, 0, 1)"> (self.name, self.gender, self.age, self.fight)
</span><span style="color: rgba(0, 0, 255, 1)">print</span><span style="color: rgba(0, 0, 0, 1)"> temp
</span><span style="color: rgba(0, 128, 0, 1)">#</span><span style="color: rgba(0, 128, 0, 1)"> ##################### 开始游戏 #####################</span>
<span style="color: rgba(0, 0, 0, 1)">
cang </span>= Person(<span style="color: rgba(128, 0, 0, 1)">'</span><span style="color: rgba(128, 0, 0, 1)">苍井井</span><span style="color: rgba(128, 0, 0, 1)">'</span>, <span style="color: rgba(128, 0, 0, 1)">'</span><span style="color: rgba(128, 0, 0, 1)">女</span><span style="color: rgba(128, 0, 0, 1)">'</span>, 18, 1000) <span style="color: rgba(0, 128, 0, 1)">#</span><span style="color: rgba(0, 128, 0, 1)"> 创建苍井井角色</span>
dong = Person(<span style="color: rgba(128, 0, 0, 1)">'</span><span style="color: rgba(128, 0, 0, 1)">东尼木木</span><span style="color: rgba(128, 0, 0, 1)">'</span>, <span style="color: rgba(128, 0, 0, 1)">'</span><span style="color: rgba(128, 0, 0, 1)">男</span><span style="color: rgba(128, 0, 0, 1)">'</span>, 20, 1800) <span style="color: rgba(0, 128, 0, 1)">#</span><span style="color: rgba(0, 128, 0, 1)"> 创建东尼木木角色</span>
bo = Person(<span style="color: rgba(128, 0, 0, 1)">'</span><span style="color: rgba(128, 0, 0, 1)">波多多</span><span style="color: rgba(128, 0, 0, 1)">'</span>, <span style="color: rgba(128, 0, 0, 1)">'</span><span style="color: rgba(128, 0, 0, 1)">女</span><span style="color: rgba(128, 0, 0, 1)">'</span>, 19, 2500) <span style="color: rgba(0, 128, 0, 1)">#</span><span style="color: rgba(0, 128, 0, 1)"> 创建波多多角色</span>
<span style="color: rgba(0, 0, 0, 1)">
cang.incest() </span><span style="color: rgba(0, 128, 0, 1)">#</span><span style="color: rgba(0, 128, 0, 1)">苍井空参加一次多人游戏</span>
dong.practice()<span style="color: rgba(0, 128, 0, 1)">#</span><span style="color: rgba(0, 128, 0, 1)">东尼木木自我修炼了一次</span>
bo.grassland() <span style="color: rgba(0, 128, 0, 1)">#</span><span style="color: rgba(0, 128, 0, 1)">波多多参加一次草丛战斗</span>
<span style="color: rgba(0, 128, 0, 1)">#</span><span style="color: rgba(0, 128, 0, 1)">输出当前所有人的详细情况</span>
<span style="color: rgba(0, 0, 0, 1)">cang.detail()
dong.detail()
bo.detail()
cang.incest() </span><span style="color: rgba(0, 128, 0, 1)">#</span><span style="color: rgba(0, 128, 0, 1)">苍井空又参加一次多人游戏</span>
dong.incest() <span style="color: rgba(0, 128, 0, 1)">#</span><span style="color: rgba(0, 128, 0, 1)">东尼木木也参加了一个多人游戏</span>
bo.practice() <span style="color: rgba(0, 128, 0, 1)">#</span><span style="color: rgba(0, 128, 0, 1)">波多多自我修炼了一次</span>
<span style="color: rgba(0, 128, 0, 1)">#</span><span style="color: rgba(0, 128, 0, 1)">输出当前所有人的详细情况</span>
<span style="color: rgba(0, 0, 0, 1)">cang.detail()
dong.detail()
bo.detail()
游戏人生</span></pre></div><span class="cnblogs_code_collapse">View Code</span></div></td></tr></tbody></table>
**二、继承**
继承,面向对象中的继承和现实生活中的继承相同,即:子可以继承父的内容。
例如:
猫可以:喵喵叫、吃、喝、拉、撒
狗可以:汪汪叫、吃、喝、拉、撒
如果我们要分别为猫和狗创建一个类,那么就需要为 猫 和 狗 实现他们所有的功能,如下所示:

class 猫: def 喵喵叫(self): print '喵喵叫'
def 吃(self): # do something
def 喝(self): # do something
def 拉(self): # do something
def 撒(self): # do something
class 狗: def 汪汪叫(self): print '喵喵叫'
def 吃(self): # do something
def 喝(self): # do something
def 拉(self): # do something
def 撒(self): # do something
伪代码
View Code
上述代码不难看出,吃、喝、拉、撒是猫和狗都具有的功能,而我们却分别的猫和狗的类中编写了两次。如果使用 继承 的思想,如下实现:
动物:吃、喝、拉、撒
猫:喵喵叫(猫继承动物的功能)
狗:汪汪叫(狗继承动物的功能)

class 动物: def 吃(self): # do something
def 喝(self): # do something
def 拉(self): # do something
def 撒(self): # do something
在类后面括号中写入另外一个类名,表示当前类继承另外一个类
class 猫(动物): def 喵喵叫(self): print '喵喵叫'
在类后面括号中写入另外一个类名,表示当前类继承另外一个类
class 狗(动物): def 汪汪叫(self): print '喵喵叫' 伪代码
View Code

class Animal: def eat(self): print "%s 吃 " %self.name def drink(self): print "%s 喝 " %self.name def shit(self): print "%s 拉 " %self.name def pee(self): print "%s 撒 " %self.name class Cat(Animal): def __init__(self, name): self.name = name self.breed = '猫'
def cry(self): print '喵喵叫'
class Dog(Animal): def __init__(self, name): self.name = name self.breed = '狗'
def cry(self): print '汪汪叫'
######### 执行
c1 = Cat('小白家的小黑猫') c1.eat()
c2 = Cat('小黑的小白猫') c2.drink()
d1 = Dog('胖子家的小瘦狗') d1.eat()
代码实例
代码实例
**所以,对于面向对象的继承来说,其实就是将多个类共有的方法提取到父类中,子类仅需继承父类而不必一一实现每个方法。**
**_注:除了子类和父类的称谓,你可能看到过 派生类 和 基类 ,他们与子类和父类只是叫法不同而已。_**
_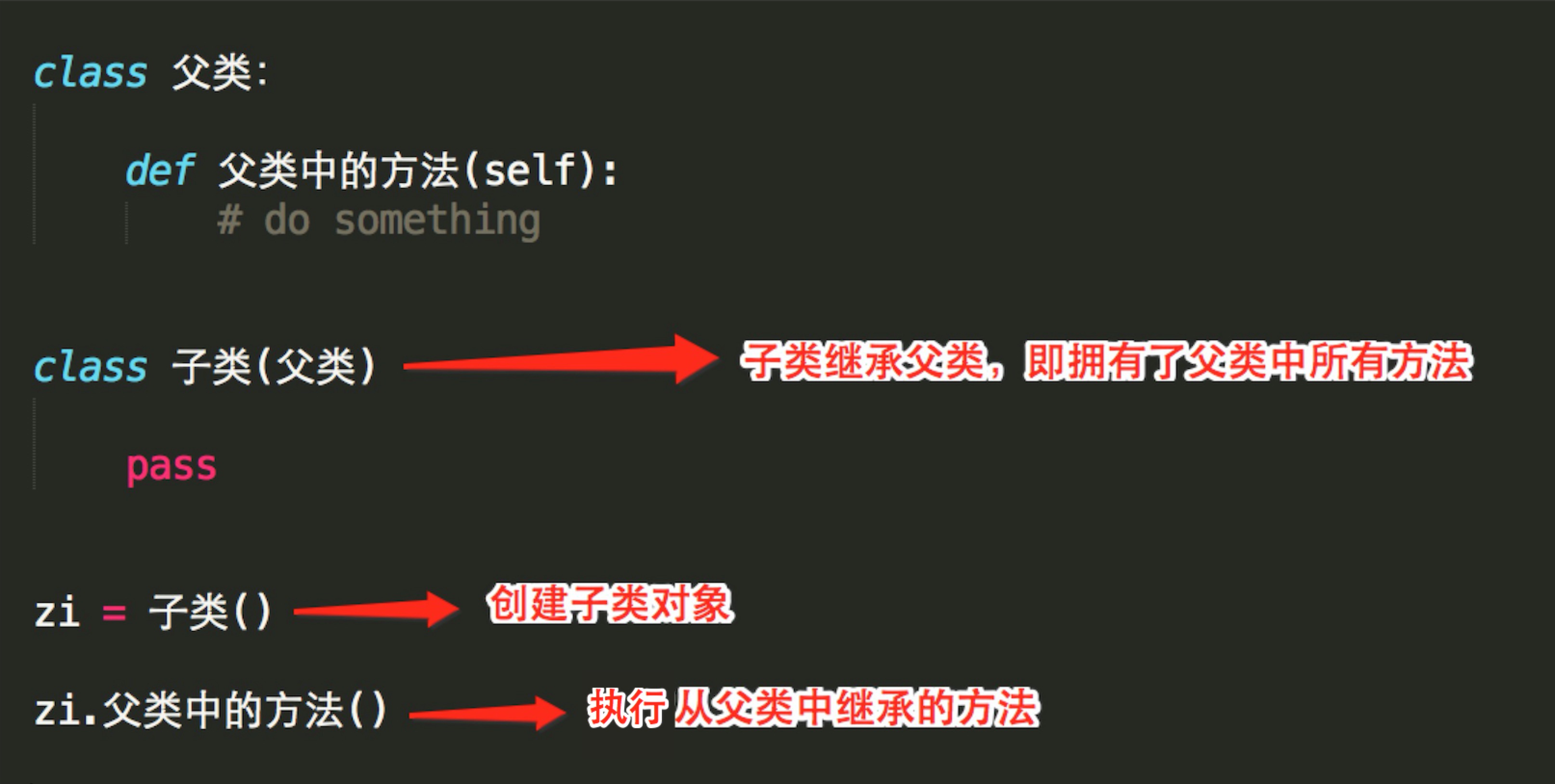_
学习了继承的写法之后,我们用代码来是上述阿猫阿狗的功能:

class Animal: def eat(self): print "%s 吃 " %self.name def drink(self): print "%s 喝 " %self.name def shit(self): print "%s 拉 " %self.name def pee(self): print "%s 撒 " %self.name class Cat(Animal): def __init__(self, name): self.name = name self.breed = '猫'
def cry(self): print '喵喵叫'
class Dog(Animal): def __init__(self, name): self.name = name self.breed = '狗'
def cry(self): print '汪汪叫'
######### 执行
c1 = Cat('小白家的小黑猫') c1.eat()
c2 = Cat('小黑的小白猫') c2.drink()
d1 = Dog('胖子家的小瘦狗') d1.eat()
代码实例
代码实例
**那么问题又来了,多继承呢?**
* 是否可以继承多个类
* 如果继承的多个类每个类中都定了相同的函数,那么那一个会被使用呢?
1、Python的类可以继承多个类,Java和C#中则只能继承一个类
2、Python的类如果继承了多个类,那么其寻找方法的方式有两种,分别是:**深度优先**和**广度优先**
****下图中B、C类继承D类,A类继承B、C类。****
**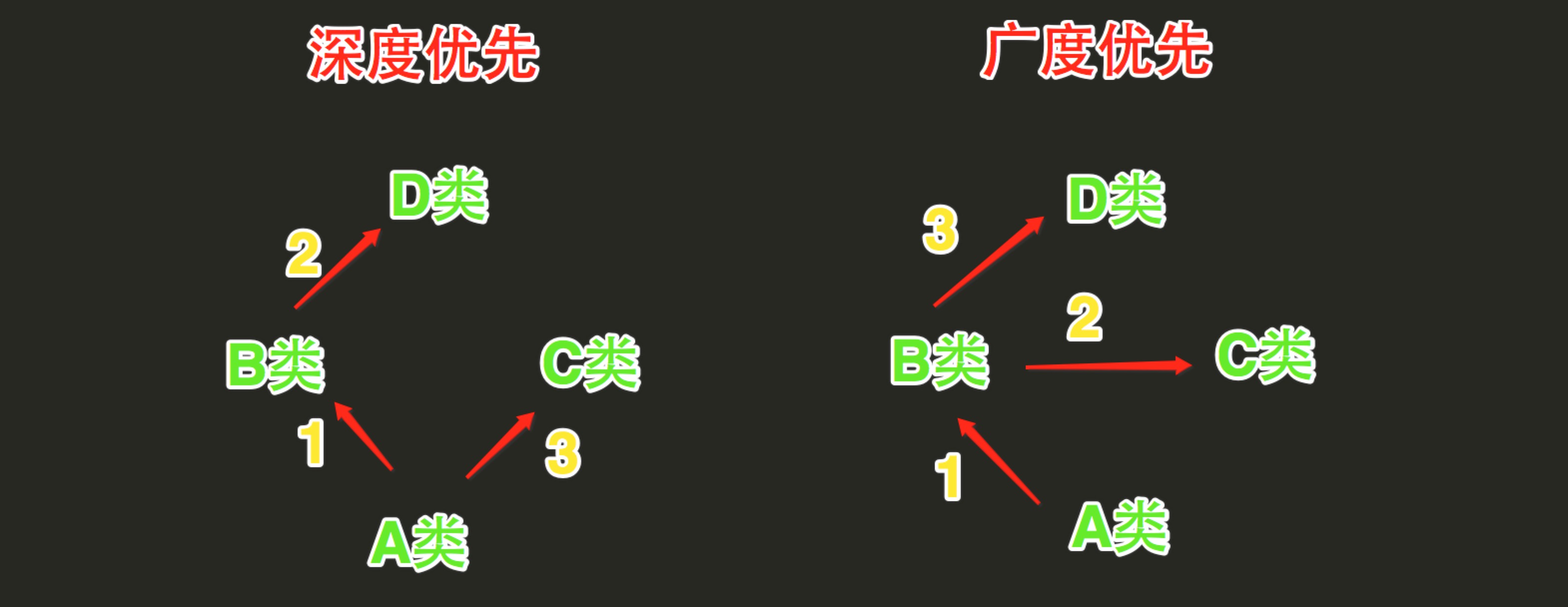**
* 当类是经典类时,多继承情况下,会按照深度优先方式查找
* 当类是新式类时,多继承情况下,会按照广度优先方式查找
经典类和新式类,从字面上可以看出一个老一个新,新的必然包含了跟多的功能,也是之后推荐的写法,从写法上区分的话,如果 **当前类或者父类继承了object类**,那么该类便是新式类,否则便是经典类。
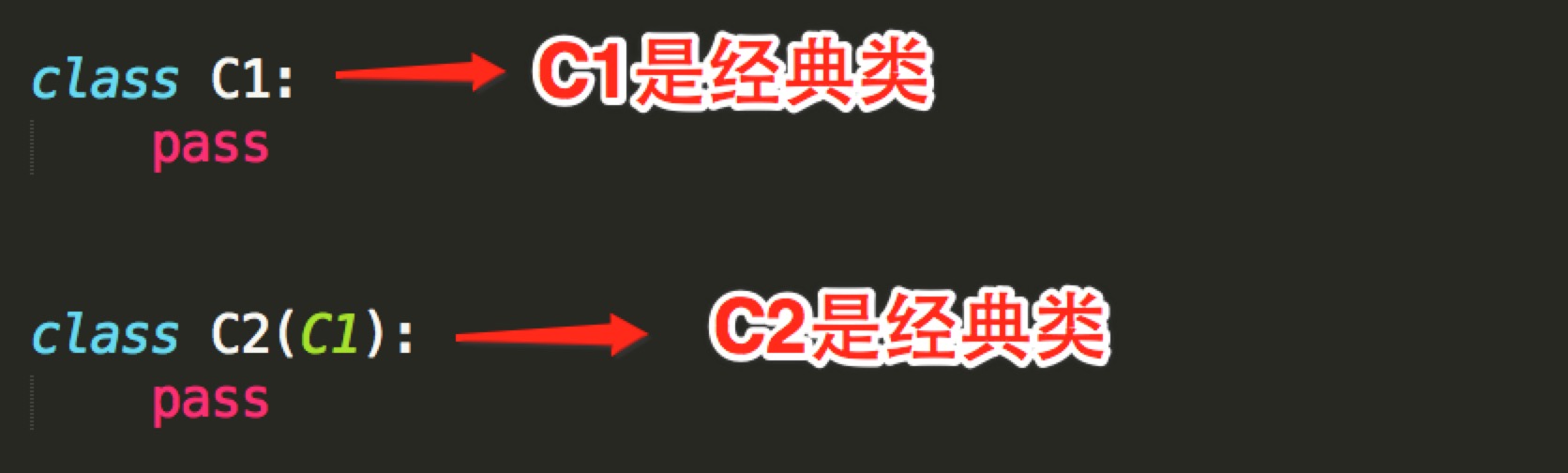 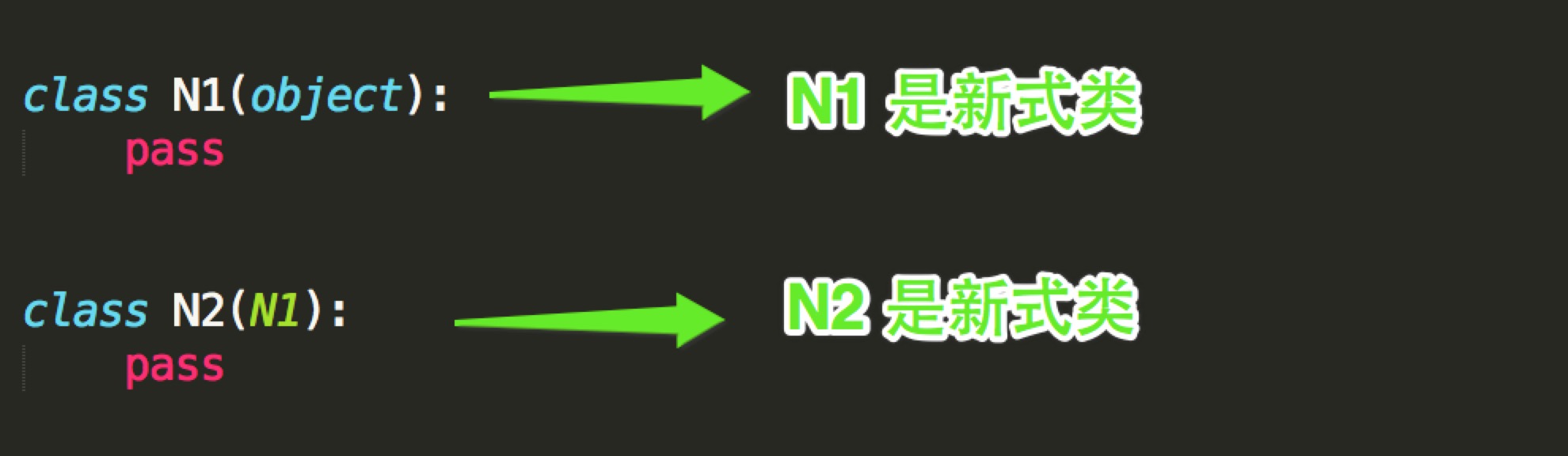

class D: def bar(self): print 'D.bar'
class C(D): def bar(self): print 'C.bar'
class B(D): def bar(self): print 'B.bar'
class A(B, C): def bar(self): print 'A.bar' a = A() # 执行bar方法时 # 首先去A类中查找,如果A类中没有,则继续去B类中找,如果B类中么有,则继续去D类中找,如果D类中么有,则继续去C类中找,如果还是未找到,则报错 # 所以,查找顺序:A --> B --> D --> C # 在上述查找bar方法的过程中,一旦找到,则寻找过程立即中断,便不会再继续找了 a.bar()
经典类多继承
经典类多继承

class D(object): def bar(self): print 'D.bar'
class C(D): def bar(self): print 'C.bar'
class B(D): def bar(self): print 'B.bar'
class A(B, C): def bar(self): print 'A.bar' a = A() # 执行bar方法时 # 首先去A类中查找,如果A类中没有,则继续去B类中找,如果B类中么有,则继续去C类中找,如果C类中么有,则继续去D类中找,如果还是未找到,则报错 # 所以,查找顺序:A --> B --> C --> D # 在上述查找bar方法的过程中,一旦找到,则寻找过程立即中断,便不会再继续找了 a.bar()
新式类多继承
新式类多继承
经典类:首先去**A**类中查找,如果A类中没有,则继续去**B**类中找,如果B类中么有,则继续去**D**类中找,如果D类中么有,则继续去**C**类中找,如果还是未找到,则报错
新式类:首先去**A**类中查找,如果A类中没有,则继续去**B**类中找,如果B类中么有,则继续去**C**类中找,如果C类中么有,则继续去**D**类中找,如果还是未找到,则报错
注意:在上述查找过程中,一旦找到,则寻找过程立即中断,便不会再继续找了
**三、多态**
Pyhon不支持Java和C#这一类强类型语言中多态的写法,但是原生多态,其Python崇尚“鸭子类型”。

class F1: pass
class S1(F1): def show(self): print 'S1.show'
class S2(F1): def show(self): print 'S2.show'
由于在Java或C#中定义函数参数时,必须指定参数的类型 # 为了让Func函数既可以执行S1对象的show方法,又可以执行S2对象的show方法,所以,定义了一个S1和S2类的父类 # 而实际传入的参数是:S1对象和S2对象
def Func(F1 obj): """Func函数需要接收一个F1类型或者F1子类的类型"""
print obj.show()
s1_obj = S1() Func(s1_obj) # 在Func函数中传入S1类的对象 s1_obj,执行 S1 的show方法,结果:S1.show s2_obj = S2() Func(s2_obj) # 在Func函数中传入Ss类的对象 ss_obj,执行 Ss 的show方法,结果:S2.show Python伪代码实现Java或C#的多态
Python伪代码实现Java或C#的多态

class F1: pass
class S1(F1): def show(self): print 'S1.show'
class S2(F1): def show(self): print 'S2.show'
def Func(obj): print obj.show()
s1_obj = S1() Func(s1_obj)
s2_obj = S2() Func(s2_obj)
Python “鸭子类型”
```
Python “鸭子类型”
总结
以上就是本节对于面向对象初级知识的介绍,总结如下:
- 面向对象是一种编程方式,此编程方式的实现是基于对 类 和 对象 的使用
- 类 是一个模板,模板中包装了多个“函数”供使用
- 对象,根据模板创建的实例(即:对象),实例用于调用被包装在类中的函数
- 面向对象三大特性:封装、继承和多态
转载地址:http://www.cnblogs.com/wupeiqi/p/4493506.html
***********************************************************
学习永远不晚。——高尔基
***********************************************************
本文转自 https://www.cnblogs.com/chengd/articles/7287528.html,如有侵权,请联系删除。